Firstly, you may wonder what is macros ?
I. Basic concepts !
1. A html element on a webpage
- For any website, you can see it's constructed by many elements. In this turorial I will use chrome inspector to view them. Open this sample page on chrome, then press F12 to view source code. As you can see, there are a lot of elements. Eg: div, button, span, ..etc.
- I will this sample page through this turorial, please have a look
2. Element's attributes
- Each element has it's own attributes. for example, look at this button element
<button onclick="ClickMe(this)" class="btn" id="btn1">button 1</button>
This button has tag name is button and it's has flowing attributes:
onclick with value ClickMe(this)
class with value btn
id with value btn1
text with value button 1
- You can find out the html code of an element on a webpage easily in chrome with just 3 simple steps
1. Click to inspector icon on chrome inspector or press Ctrl Shift C
2. Click to the element you want to inspect
3. Look at the result (the element would be highlighted)
3. 9Hits Macros - common commands
- await Delay (n): delay for n miliseconds
- Random(start, end): Generate a random integer number between start - end
- Click commands: ClickById, ClickByXpath, ClickByClass, ClickByTag.
- GenerateXpath: Create xpath for an element.
- Operators: Arithmetic (+, -, *, /), Relational (==, !=, <, <=, >, >=), Logical (&&, ||, !)
- Loops
+ Syntax
while(condition){
statement(s);
}
+ Examlple
a = 10;
while( a < 20 ) {
txt = "value of a: " + a;
Alert(txt);
a = a+1;
}
- Decision making
+ Syntax
if(boolean_expression 1) {
// Executes when the boolean expression 1 is true
}else if( boolean_expression 2) {
// Executes when the boolean expression 2 is true
}else if( boolean_expression 3) {x
// Executes when the boolean expression 3 is true
} else {
// Executes when the none of the above condition is true.
}
For full list of commands, please access the Using Macros session from 9hits panel
II. Practice
1. Macro editor
- Macro Editor is a tool that help you to testing your script before put it into work on 9Hits Traffic Exchange system. Open it from 9Hits Viewer
- Every command must end with semi-colon (;)
- URL field is the link of the website that you want macros run on. other fields are optional
2. Make a simple click
- I will use the sample page. Let say now I want to click to the button 1.
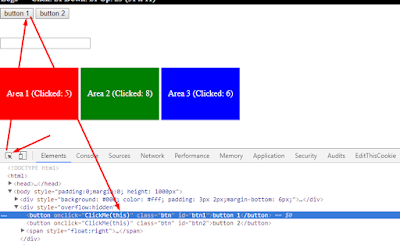
It's look like this
<button onclick="ClickMe(this)" class="btn" id="btn1">button 1</button>
As you can see, this element has id="btn1". So I can just simple use await ClickById("btn1");. And an important thing that we need ensure the page has loaded before the click command execute. delay for a while should be good. assuming I delay for 10s before click. The script should be
await Delay(10000);
await ClickById("btn1");
Let try it on your macro editor, you may need to modify the Delay value if the site need more time to load completely.
An other option, this button has class is "btn", we have 2 buttons with class = "btn" (button 1, button 2), and you can use await ClickByClass("btn");. By default, the ClickByClass command would click the first element that matched the class name ("btn"). If you want to click to the 2nd element which has class btn. The command should be await ClickByClass("btn", 1); (element position is zero base, so 0 is the first, 1 is the 2nd and so on).
If you want to click random any button has class = "btn". Just use await ClickByClass("btn", "random");
ClickByTag is nearly the same. you may use await ClickByTag("button", 1) to click to the 2nd button, or await ClickByTag("button", "random") to click any button randomly. Don't forget the Delay command before click.
3. GenerateXpath and ClickByXpath.
Combining there 2 command would let you make a click flexibly. Look at the top right button.
<button id="skip" style="display:inline-block" onclick="ClickMe(this)">please click me guy</button>
Let say I want to click to the button has text equals to please click me guy.
First, I will create xpath to target to that button, then pass the generated xpath to the ClickByXpath command
await Delay(15000);
theXpath = GenerateXpath("button", "text", "please click me guy");
await ClickByXpath(theXpath);
Explanation:
- delay for 15s to wait for page load.
- theXpath: Just is name of variable, you may naming any name you want
- In GenerateXpath command, button is the tag name, text is the attribute and the text "please click me guy" is the value to compare.
- pass the generated xpath to the ClickByXpath command to do click.
Ok, now you know GenerateXpath("button", "text", "please click me guy") mean target to the button has text equals to please click me guy.
There are 3 other options, please pay attention with the use of % sign
- GenerateXpath("button", "text", "please click%"). Target the button has text starts with please click... (so if the button has text is please click him, please click her, please click bla bla also work)
- GenerateXpath("button", "text", "%click me guy"). Target the button has text ends with click me guy.
- GenerateXpath("button", "text", "%click me%"). Target the button has text contains click me.
You may now try on macro editor.
You can use any attribute to generate xpath.
<button id="skip" style="display:inline-block" onclick="ClickMe(this)">please click me guy</button>
If I dont want to use text attribute. I can use other attribute, e.g. style attribute.
GenerateXpath("button", "style", "display:inline-block");
GenerateXpath("button", "style", "display:inline%");
GenerateXpath("button", "style", "%inline-block");
GenerateXpath("button", "style", "%inline-%");
The script should be
await Delay(15000);
theXpath = GenerateXpath("button", "style", "display:inline-block");
await ClickByXpath(theXpath);
You may also pass the GenerateXpath directly to the ClickByXpath command
await Delay(15000);
await ClickByXpath(GenerateXpath("button", "style", "display:inline-block"));
=> Got it ? Try on macro editor now !
4. Click to an element by rate
Look at the sample page, we have 3 areas here
I want macro click to Area 1, but not click all the time, just click about 20%, and 80% do nothing.
The logic: I will generate a random number between 0 - 100. If this number less than 20 so do the click, else do nothing. look like this
the range
[0--- click --20-----------------------do no thing ------------------100]
Let script it
await Delay(15000);
randNum = Random(0, 100);
if (randNum < 20)
{
await ClickById("area1");
}
So the click only execute if the randNum < 20.
Done !.
Now, I want to click to the area1 about 20%, area2 50% and area3 30%.
The logic is different a bit, Im still create a random number in range [0-100].
if the the random number < 20 click area1, 20 - 70 click area 2, and greater than or equals to 70 click area 3.
the range
[0--- area1--20------------------area2----------------70------area3-----100]
randNum = Random(0, 100);
if (randNum < 20)
{
await ClickById("area1");
}
else if(randNum >=20 && randNum <70)
{
await ClickById("area2");
}
else if(randNum >= 70)
{
await ClickById("area3");
}
For click command, you may use click by class, click by xpath or any other click command as it target to exact the element that you want to click.
Try it on macro editor !
5. Set value to the textbox
We have one textbox here
<input type="text" class="textfield" id="typetome" />
There are some SetBy.. commands you can found in 9hits panel.
I will use SetById (as this element has id is typetome).
await Delay(10000);
SetById("typetome", "value", "hello");
Or to more like human do. I will click to the text box, and then typing the "hello" text.
await Delay(3000); await ClickById("typetome");
await Delay(2000); await Typing("hello");
Let try it on macro editor !
Tip: you can delay for a random time, like await Delay(Random(15000, 20000));
III. Put it into work
- Once you have tested your macro, now its time to put it to work, on your site setting on 9hits panel, just put your macro to the macro options. While other users in the system viewing your site, your macro will also be executed.
- Please note if you set the option Allow Old Version to Yes, so the macro would not run on the old version. You may need to rewrite your macro and put it into the Legacy tab to make it compatible with the viewer v1. In most case, if your macro is not so complex, just remove await keywork to get it compatible with v1.
- Remember: Not all the running viewers on system has same connection (speed) like your local computer, so it's may take more time to load your website, it's mean you may need to delay longer than your test to ensure macro run properly.
Eg. your tested script is
await Delay(10000);
await ClickById("btn1");
But when you put it into work. You should delay for longer time, may be 15 or 20s.
await Delay(20000);
await ClickById("btn1");
On next post, I will show you how to search on google for a special keywords and looking for the result on a limit of pages.
=> http://blog.9hits.com/2018/01/google-searches-for-keyword-within.html
Please leave any comment here or contact us if you need help !
Ok thank you for this tutorial it's very clear. But clicking a button is fine but if I want to click on an iframe I have to do how?
ReplyDeleteHi, if the iframe has ID, just use ClickById, or you may use ClickByXpath. may be like this
DeleteClickByXpath(GenerateXpath("iframe", "src", "http://blabla.com/xxx"));
Thanks for your feedback. But it's not so simple. When you have an Iframe created by a Javascript script that contains a html page with head and body tag as you can do?
ReplyDeletejust create a div with unique id, then put your javascript ad code inside it. Final, use ClickByID or other same else
DeleteHi Faucetbtc! Did you try on macro editor, if your script doesnt work, please contact me via skype or support from 9hits panel :)
DeleteThis comment has been removed by the author.
ReplyDeleteWhy is this? This is bunch of confusion. Oh my god: i could not understand anything. If any one want to buy service of 9hits he has to understand all these confusion. Please explain without confusion
ReplyDeleteHi, this is just a macros part, without this, it just like other traffic exchange system. It's not hard to do, just try it with macro editor. And we always ready to help :)
DeleteDonde encuentro el editor de macros?
Deletehi I have a problem with shorte.st I want a macro pleaze,you note that i have a premiem account please help me
ReplyDeletehi, its already shared here
Deletehttps://forum.9hits.com/viewtopic.php?f=7&t=23
thanks
ReplyDeleteMacro click backlink to backl primary site.
ReplyDeleteawait delay(6000);
tam=GenerateXPath("a","href","your url");
ClickByXPath(tam);
I don`t want open new tab, so i want only run 1 tab. Can you help me
plz help me i want macro or tutoriel of shorte.st
ReplyDeleteIf I want to creat a macro like this
ReplyDeletescroll_to => 0.124
sleep => 1
scroll_to => 0.04
sleep => 3
click_position_range => 101,158 => 657,367
Can u translate to your macro language ???
It's easy. You may use like this
Deleteawait Delay(10000); //delay 10s, we may need to wait for page load
ScrollTo(0, 124); //scroll down 124 px
await Delay (1000); //delay for 1s
//..... you may scroll or delay more
ClickByCoordinates(101, 158, 657, 367);
That's all. It's even easy like your pseudo code :)
Hello,
ReplyDeleteI have a problem with automatic clicking code anywhere on my site for popunder
macro does not work and i got no profit
ReplyDeletei want to click on 1 anchor text , how can i do ?
ReplyDeleteBuen día.
ReplyDeleteRequiero eliminar el atributo disabled de un objeto del formulario. ¿Cómo lo puedo hacer?
i has add link and copy macros but i not received viewer, url http//google.com, but apllication not send macros
ReplyDeleteGreat tutorials. It gives a detailed instruction for us being a newbie. keep on sharing. 토토사이트
ReplyDeletecan we do a click on google ad via any code?
ReplyDelete